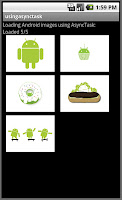
Add into your AndroidManifest.xml this permission:
main.xml
main.java
package com.blog.usingasynctask; import java.io.IOException; import java.io.InputStream; import java.net.MalformedURLException; import java.net.URL; import android.app.Activity; import android.content.Context; import android.graphics.drawable.Drawable; import android.os.AsyncTask; import android.os.Bundle; import android.widget.ImageView; import android.widget.TextView; public class main extends Activity { final String[] links = { "http://www.android.com/media/wallpaper/gif/android_logo.gif", "http://www.android.com/media/wallpaper/gif/cupcake_2009.gif", "http://www.android.com/media/wallpaper/gif/donut_2009.gif", "http://www.android.com/media/wallpaper/gif/eclair_2009.gif", "http://www.android.com/media/wallpaper/gif/androids.gif" }; TextView count; int id; /** Called when the activity is first created. */ @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); id = 0; count = (TextView) findViewById(R.id.textView2); startDownload( id ); } public void startDownload(int id){ // calling the asynchronous task new ImageLoadingTask().execute(); }; public void displayImage(Drawable image){ ImageView iv = null; switch (id){ case 0 : iv = (ImageView) findViewById(R.id.imageView1); break; case 1 : iv = (ImageView) findViewById(R.id.imageView2); break; case 2 : iv = (ImageView) findViewById(R.id.imageView3); break; case 3 : iv = (ImageView) findViewById(R.id.imageView4); break; case 4 : iv = (ImageView) findViewById(R.id.imageView5); break; } iv.setImageDrawable(image); id++; if (id < 5) new ImageLoadingTask().execute(); }; public class ImageLoadingTask extends AsyncTask{ @Override protected void onPreExecute(){ count.setText( count.getText() + " ... in progress image "+ id+1 ); } @Override protected Drawable doInBackground(Void... params) { //write here code that can take a long time Drawable image = ImageOperations(getApplicationContext() ,links[id], id +".gif"); //publishProgress calls onProgressUpdate to update somthing on UI publishProgress(); return image; } @Override // Call this method when doInBackground is working to update the progress protected void onProgressUpdate(Void... values) { int number = id+1; count.setText("Loaded " + number + "/5"); } @Override protected void onPostExecute(Drawable result) { // update the UI with loaded information displayImage(result); } // Additional method to load images from web private Drawable ImageOperations(Context ctx, String url, String saveFilename) { try { URL imageUrl = new URL(url); InputStream is = (InputStream) imageUrl.getContent(); Drawable d = Drawable.createFromStream(is, "src"); return d; } catch (MalformedURLException e) { e.printStackTrace(); return null; } catch (IOException e) { e.printStackTrace(); return null; } } } }